Commit Stomping
Manipulating Git Histories to Obscure the Truth
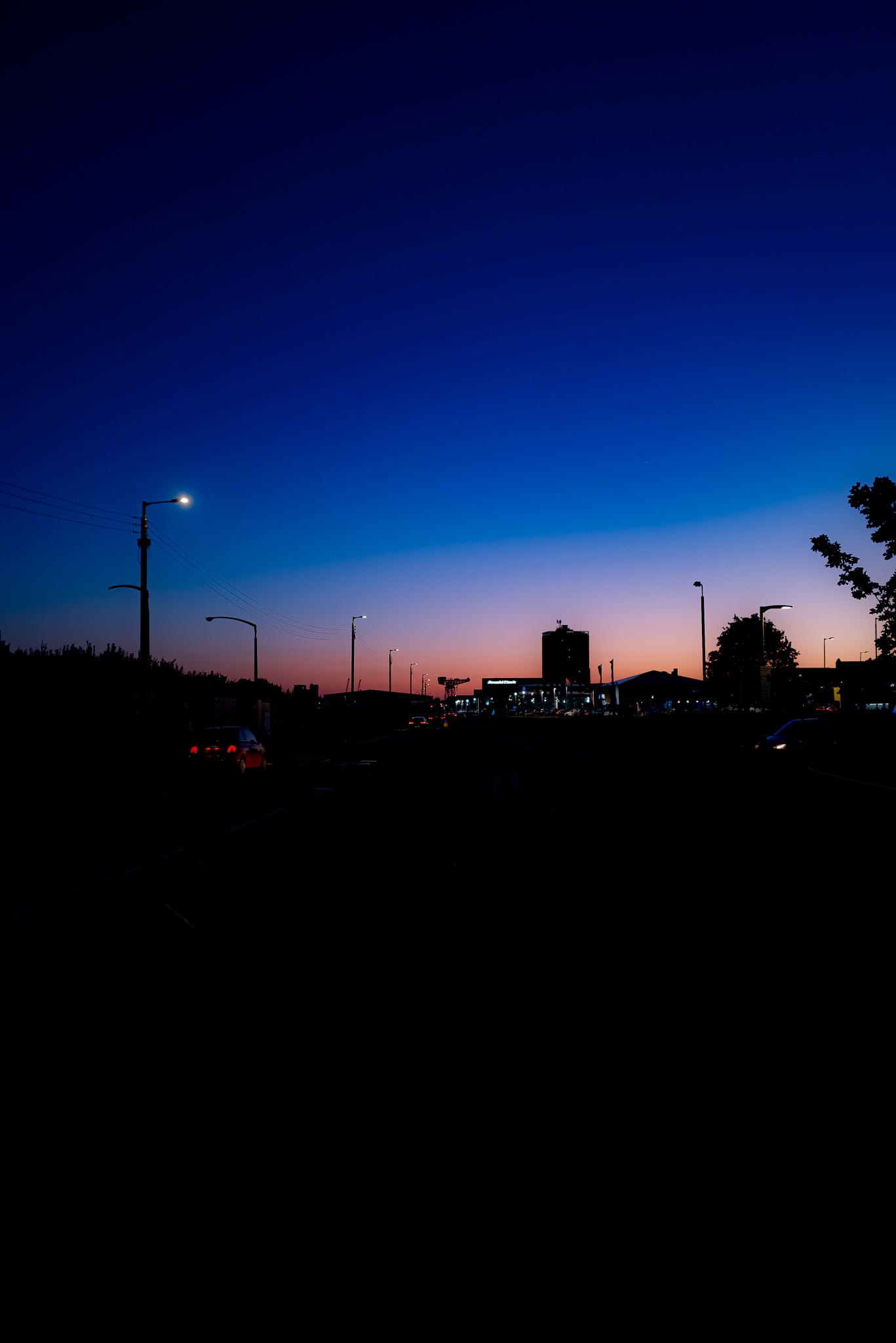
Commit Stomping is a technique inspired by timestomping, a well-known method used in offensive operations where file metadata is manipulated to hide the true timing of actions. In Git, Commit Stomping involves altering commit timestamps to mislead observers about when changes were introduced.
This is not a bug or vulnerability in Git. It is a feature that, when misused, can be leveraged to disguise malicious or unauthorised changes, making it harder to establish an accurate timeline during audits, investigations, or code reviews.
I have written/researched the topic before when I wrote RepoMan and the accompanying blog post looking at ways to commit code influenced by AI but never explored the topic fully of a standalone technique.
If you decide to copy this post word for word PLEASE attribute it to this post rather than blind copy and scrape.
Why This Technique Matters
Git commit history is often relied upon as a source of truth in:
- Incident response and forensic timelines
- Code auditing and compliance reviews
- Attribution in team or open-source environments
When commit timestamps are falsified, it becomes more difficult to determine the sequence of changes and their origin. This has significant implications in the context of software supply chain security, insider threats, and long-term audit trails.
How Git Tracks Time
Git doesn’t just store what changed. It also stores metadata about who made the change and when. Each commit includes two distinct timestamps:
GIT_AUTHOR_DATE
: the date and time when the content was originally writtenGIT_COMMITTER_DATE
: the date and time when the commit was finalised into the repository
In most workflows, these values are identical. However, differences arise when rebasing, amending commits, or when someone other than the original author performs the commit.
Rebase and Amend Scenarios
Using git rebase
or git commit --amend
rewrites commits. Git retains the GIT_AUTHOR_DATE
but updates the GIT_COMMITTER_DATE
to reflect when the change was rewritten.
git commit --amend
This adjusts the commit metadata without changing the authored time unless explicitly overridden.
Multiple Committers
In collaborative workflows:
- A developer may create a patch and email it
- A maintainer or reviewer may then commit it
In these cases, the author and committer can differ in identity and timezone. This divergence can complicate forensic analysis if timestamps are not normalised.
Manual Timestamp Control
Git allows you to control both timestamps through environment variables:
GIT_AUTHOR_DATE="2025-05-01T10:00:00" \
GIT_COMMITTER_DATE="2025-01-15T15:30:00" \
git commit -m "Insert logic for token generation"
This enables legitimate metadata preservation, or misuse to forge history.
Supported Timestamp Formats
Git supports various formats for these environment variables and the --date
flag:
1. Git Internal Format
<unix timestamp> <time zone offset>
Example:
GIT_AUTHOR_DATE="1700000000 +0100"
This represents 14 November 2024, 06:13:20 CET.
2. ISO 8601
GIT_AUTHOR_DATE="2024-11-14T06:13:20+01:00"
GIT_AUTHOR_DATE="2024-11-14 06:13:20 +0100"
3. RFC 2822
GIT_AUTHOR_DATE="Tue, 14 Nov 2024 06:13:20 +0100"
4. Relative
GIT_AUTHOR_DATE="2 weeks ago"
GIT_COMMITTER_DATE="yesterday"
5. Epoch
GIT_AUTHOR_DATE="@1700000000"
Equivalent to "1700000000 +0000" unless specified.
Committing with Custom Timestamps
Git allows you to assign arbitrary timestamps to your commits at the time of creation. This means you can forge commit dates to appear as if the work happened at a completely different point in time. This can be useful for legitimate reasons such as porting historical code into a new repo while preserving authoring dates, but it’s also the basis of Commit Stomping when used maliciously.
To commit with backdated timestamps, you can explicitly set both the author and committer dates using environment variables:
GIT_AUTHOR_DATE="2025-01-01T10:00:00" \
GIT_COMMITTER_DATE="2025-01-01T10:00:00" \
git commit -m "Backdated logic insert"
This will create a commit that looks like it was written and committed at the start of 2025, regardless of the actual date it was made. From a timeline analysis perspective, this is indistinguishable from a commit genuinely made at that time unless verified through external logs or mirrored infrastructure.
Rewriting History with Commit Stomping
If a commit has already been made with a current or unwanted timestamp, you can still go back and alter it. Git’s powerful history rewriting tools make it trivial to falsify timestamps after the fact. This is where Commit Stomping becomes most effective; rewriting existing commits to plant or hide actions within a fabricated historical context.
Interactive Rebase
This method is ideal for changing one or a few recent commits in a controlled way:
git rebase -i HEAD~3
This will open an editor showing the last three commits. Change pick
to edit
for the commit you want to alter. Then:
GIT_COMMITTER_DATE="2025-01-01T10:00:00" \
git commit --amend --no-edit --date "2025-01-01T10:00:00"
git rebase --continue
This replaces the original timestamp with your chosen date while keeping the commit content and hash altered (since Git hashes include metadata). This is ideal for surgical stomping during a cleanup or manipulation campaign.
Using git filter-branch
If you're rewriting timestamps across a broader set of commits, or you want to target specific hashes deep in history, git filter-branch
allows for scripted, large-scale rewriting.
git filter-branch --env-filter '
if [ "$GIT_COMMIT" = "abc123" ]; then
export GIT_AUTHOR_DATE="2025-01-01T10:00:00"
export GIT_COMMITTER_DATE="2025-01-01T10:00:00"
fi
' -- --all
This selectively changes timestamps for commit abc123
across all branches. You can expand the logic to match on author names, messages, or ranges. While powerful, filter-branch
is also resource-heavy and irreversible without backups.
Consider using
git filter-repo
instead. It’s faster, more flexible, and safer for large repositories.
Automating Commit Stomping
If the goal is to hide the real order of changes or make a repository’s timeline look noisy and random, you can automate Commit Stomping across the entire commit history. This introduces entropy into the timeline, making it much harder to identify when key actions took place.
Here’s a simple example using filter-branch
and randomised Unix timestamps:
git filter-branch --env-filter '
export GIT_AUTHOR_DATE="$(date -d @$((RANDOM % 1000000000 + 1000000000)))"
export GIT_COMMITTER_DATE="$GIT_AUTHOR_DATE"
' -- --all
This script randomly sets both the author and committer dates to values within a wide epoch range. You can tune the randomness or target specific ranges to blend changes into specific timeframes (e.g., placing backdoors into old release cycles).
This approach is noisy, irreversible, and a clear sign of tampering when done without reason but in adversarial simulations or offensive operations, it can completely break timeline-based investigations.
Detecting Commit Stomping
Spotting Commit Stomping isn’t always straightforward, especially in fast-moving repositories or those without consistent commit hygiene. However, there are clear indicators that should raise eyebrows during forensic reviews, audits, or general source control hygiene checks.
Look for the following:
-
Identical or overly similar timestamps across multiple commits
Commits with the exact sameGIT_AUTHOR_DATE
andGIT_COMMITTER_DATE
, especially in succession, suggest automation or manual backdating. This is particularly suspicious when the commits appear to reflect logically separate pieces of work. -
Mismatched author and committer dates
If the author date is significantly older than the committer date (e.g., by months or years), it may indicate a rewritten or forged history. While some discrepancy is normal during rebases or patch applications, large gaps should be investigated. -
Chronological inconsistencies
Commits that appear out of sequence where a newer commit has an earlier timestamp can be a sign of history manipulation. While Git does not enforce strict chronological order, the pattern of such commits can reveal tampering or signs of nefarious actions at play. -
Discrepancies with external logs
Compare commit timestamps with your CI/CD pipelines, webhook event logs, or mirrored repositories. If a commit claims to be from January but was first seen by the build system in April, that’s a red flag. -
Unusual clusters of historical backdating
If a contributor suddenly starts committing large volumes of code with timestamps from the distant past, it could be an attempt to insert or bury changes without drawing attention.
Regularly auditing commit metadata especially in regulated or high-trust environments is a valuable part of supply chain integrity.
Defending Against Commit Stomping
Git is a distributed system designed around flexibility and offline workflows. This flexibility includes letting users assign arbitrary timestamps to commits, which also means Git does nothing to verify whether the provided dates are genuine. If you're looking to harden a repository against timeline manipulation, the following measures will help:
-
Require and verify signed commits
GPG or SSH-signed commits provide a way to validate the identity of the committer. While this doesn't prevent timestamp tampering directly, it adds a layer of accountability and helps detect unauthorised rewrite attempts. Integrate signature checks into your CI pipelines and reject unsigned commits on protected branches. -
Capture metadata on ingest
Log commit events at the point they enter your ecosystem this could be a timestamped record in CI/CD pipelines, audit trails in your Git server, or webhook payloads stored in a log aggregator. These ingestion logs create an external reference point for when changes actually occurred. -
Use mirrored repositories with immutable storage
Push commits to a read-only mirror hosted in a secure location. This mirror acts as an independent copy of the timeline. If someone rewrites history in the main repository, you can compare against the mirror to identify divergence. -
Lock down history rewriting
Disable force pushes on main or protected branches. This won't stop someone from rewriting history in a fork or personal branch, but it prevents unauthorised timeline changes from making it into your critical branches without notice. -
Monitor for anomalous patterns
Use commit analytics tools or basic scripting to watch for timestamp anomalies, such as large date gaps, backward jumps in commit history, or bursts of old-dated commits. Pair this with regular manual reviews during release or compliance cycles.
Ultimately, defending against Commit Stomping is about building layers of trust and validation around your development processes. Git gives you the rope whether teams use it to build safely or hang themselves depends on how those controls are implemented.
Final Thoughts
Commit Stomping isn’t a vulnerability in the traditional sense there’s no exploit, no CVE, and no patch incoming. It is a genuine case of it is not a bug but it is a feature of Git’s distributed and trust-based design that can be turned into a technique for deception. The issue isn’t that Git is broken, but that it trusts the user implicitly. That trust can be abused.
The ability to manually set or rewrite commit timestamps isn’t inherently bad. It is often used for practical reasons, like preserving dates when importing old projects, aligning contributions with an external system, or tidying up messy commit history. But in the wrong hands, it can be abused to rewrite the past, masking when changes were really made, hiding malicious edits, or throwing off anyone trying to trace what happened and when.
In a world where source control is part of the security perimeter, assuming the integrity of the Git log is no longer good enough. It’s important to treat version history as mutable unless proven otherwise, and to surround Git with controls that provide external validation: CI/CD logging, signed commits, immutable mirrors, and behavioural monitoring.
If you are simulating advanced adversaries, responding to insider threats, reviewing code history during an incident, or building hardened dev pipelines, the ability to manipulate timestamps must be part of your threat model and being aware of the implications of the types of attacks out there is key. If you don't look for it, you won't see it and if you're not defending against it, you're leaving a quiet gap open in your forensic trail.
At the end of the day, Git tells a story. Commit Stomping lets you rewrite the timeline of that story and change some of the key details along the way.